If you’ve worked with AWS (Amazon Web Services) and the Boto3 library in Python, chances are you’ve encountered the dreaded botocore.exceptions.NoCredentialsError: Unable to locate credentials
. This error can be frustrating, especially when you’re in the middle of a project and everything grinds to a halt. However, don’t worry! In this guide, we’ll break down the root causes of this issue, show you how to resolve it, and provide best practices to avoid it in the future.
What is Boto3 and Botocore?
Before diving into the error, let’s understand what Boto3 and Botocore are.
What is Boto3?
Boto3 is the official AWS SDK for Python, making it easy to integrate Python applications with AWS services like S3, EC2, DynamoDB, and more. Boto3 handles interactions with AWS services through API calls, and it simplifies much of the complexity that developers would face if they were to work directly with AWS REST APIs.
What is Botocore?
Botocore is the underlying library for Boto3. It provides the low-level functionality that Boto3 builds on. It handles the HTTP connections, request signing, retries, and error handling for AWS API requests. If you encounter any errors in your AWS requests through Boto3, Botocore is usually responsible for raising the exceptions.
Understanding the ‘NoCredentialsError’
The error message botocore.exceptions.NoCredentialsError: Unable to locate credentials
is quite explicit—it’s telling you that Boto3 or Botocore was unable to find the necessary AWS credentials to authenticate your requests.
AWS uses these credentials (access key and secret key) to verify that you have permission to perform the requested operations. Without these credentials, any request to an AWS service will be denied, leading to this error.
Read Also: No ‘Access-Control-Allow-Origin’ Header is Present on the Requested Resource: The Ultimate Guide

Common Causes of ‘NoCredentialsError’
There are several reasons why this error might occur. Let’s explore them.
1. Missing AWS Credentials Configuration
One of the most common causes is simply that the credentials have not been configured. Boto3 looks for credentials in specific locations. If none of these are configured, you will get the NoCredentialsError.
2. Incorrectly Set Environment Variables
Environment variables are one of the common ways to configure AWS credentials, but they must be correctly set. Incorrect spelling or paths can lead to Boto3 not finding the credentials.
3. Misconfigured AWS CLI
If you’re relying on the AWS CLI (Command Line Interface) to manage your credentials, but it’s not correctly configured, Boto3 will be unable to access them.
4. Role Misconfiguration in EC2
When using an EC2 instance with an attached IAM role, Boto3 should automatically retrieve the necessary credentials. However, if the role is misconfigured or not assigned to the instance, the credentials won’t be found.
5. Incorrect IAM Permissions
Even if the credentials are found, if the associated IAM user or role does not have the right permissions, the system may still raise a NoCredentialsError because it cannot authenticate properly.
How to Fix ‘NoCredentialsError’
Now that we’ve identified the possible causes, let’s look at how to fix this error step by step.
1. Set Up AWS Credentials via Configuration File
The most straightforward way to provide AWS credentials to Boto3 is by configuring them in the AWS credentials file.
Location of Credentials File:
By default, Boto3 looks for the credentials in a file located at ~/.aws/credentials
on Linux and macOS, or C:\Users\USERNAME\.aws\credentials
on Windows.
Structure of the Credentials File:
[default]
aws_access_key_id = YOUR_ACCESS_KEY
aws_secret_access_key = YOUR_SECRET_KEY
Ensure that this file exists and has the correct values.
2. Use Environment Variables
You can also provide AWS credentials through environment variables. These variables are:
AWS_ACCESS_KEY_ID
AWS_SECRET_ACCESS_KEY
On Linux or macOS, you would set them in your terminal as follows:
export AWS_ACCESS_KEY_ID=YOUR_ACCESS_KEY
export AWS_SECRET_ACCESS_KEY=YOUR_SECRET_KEY
For Windows, you would use:
set AWS_ACCESS_KEY_ID=YOUR_ACCESS_KEY
set AWS_SECRET_ACCESS_KEY=YOUR_SECRET_KEY
Ensure that the variables are correctly set by printing them out with echo
or printenv
.
3. Use the AWS Command Line Interface (CLI) to Configure Credentials
If you have the AWS CLI installed, you can use it to configure your credentials:
eaws configure
This will prompt you to enter your aws_access_key_id
, aws_secret_access_key
, and region. Boto3 will automatically pick up these credentials when interacting with AWS services.
4. Use IAM Roles with EC2
If you’re running your code on an EC2 instance, ensure that the correct IAM role is attached to the instance. The role should have the necessary permissions for the actions you want to perform.
To check if the role is properly attached:
- Go to the EC2 dashboard.
- Select your instance.
- Under “Actions”, click on “Modify IAM Role”.
- Ensure that the correct role is attached.
Boto3 will automatically fetch the credentials if the role is properly configured.
5. Use Session Objects
Sometimes, it’s better to manage your credentials explicitly within your Python code by using session objects. For example:
import boto3
session = boto3.Session(
aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_KEY'
)
s3 = session.resource('s3')
This way, you are providing the credentials directly to the session and bypassing the automatic credential lookup process.
Read Also: Coffee Manga: Blend Culture and Art

Best Practices for Managing AWS Credentials
Now that we’ve covered the causes and fixes, let’s talk about best practices when managing your AWS credentials.
1. Avoid Hardcoding Credentials
Never hardcode your credentials directly into your code. This practice is risky because if your code is exposed (e.g., via a public GitHub repository), your AWS account could be compromised.
2. Use IAM Roles Where Possible
Whenever you’re working with EC2 instances, Lambda functions, or other AWS services that support roles, prefer IAM roles over manually managing credentials. Roles provide a secure, automatic way to handle permissions and avoid credential leakage.
3. Use AWS Secrets Manager
For applications that need to store sensitive information such as API keys, secrets, or credentials, AWS Secrets Manager is an excellent service. It securely stores your secrets and allows you to programmatically retrieve them without hardcoding them into your application.
4. Use Multi-Factor Authentication (MFA)
Enable MFA for IAM users to add an extra layer of security. This ensures that even if your credentials are compromised, unauthorized users would still need to complete an MFA challenge.
FAQs
1. What are AWS credentials?
AWS credentials are the access key
and secret key
that allow your application to authenticate with AWS and use its services.
2. Where does Boto3 look for credentials by default?
Boto3 looks for credentials in the following order: environment variables, AWS credentials file, AWS CLI configuration, and instance metadata if running on EC2.
3. How do I avoid the ‘NoCredentialsError’ on EC2 instances?
Ensure that the correct IAM role is attached to your EC2 instance, and the role has the necessary permissions for your application.
4. Can I use AWS Secrets Manager to store my credentials?
Yes, AWS Secrets Manager is a secure way to store and manage credentials, API keys, and other sensitive information.
5. Why should I avoid hardcoding credentials in my code?
Hardcoding credentials in your code is a security risk. If your code is exposed, attackers could gain access to your AWS account and services.
Read Also: King Von Autopsy : The Rise, Tragedy, and Enduring Legacy of a Hip-Hop Icon
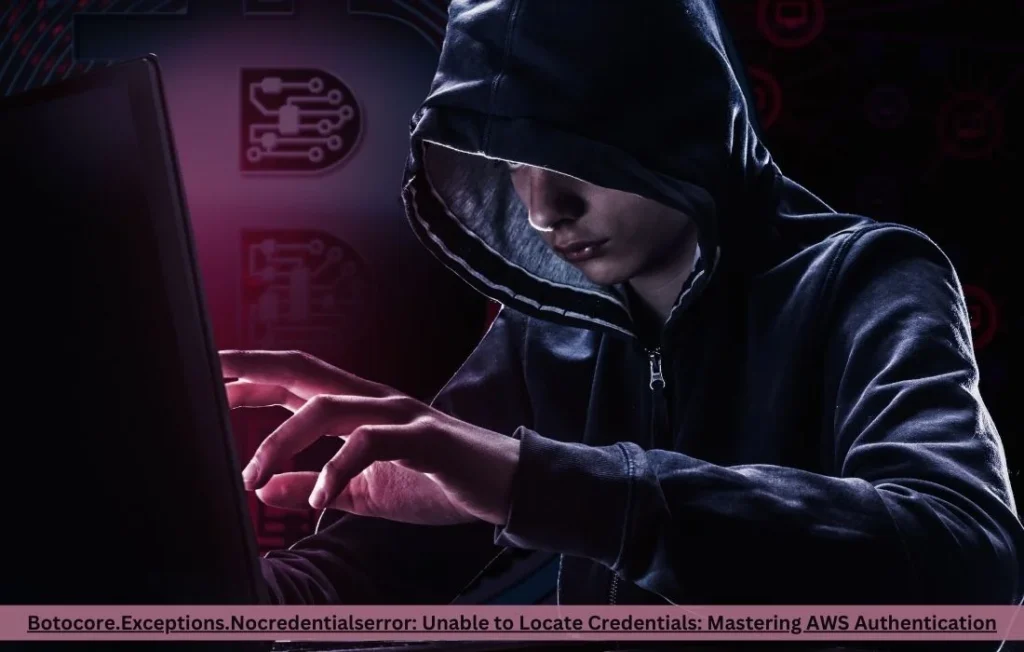
Conclusion
The botocore.exceptions.NoCredentialsError: Unable to locate credentials
error is a common hurdle when working with AWS and Python’s Boto3 library. Thankfully, it’s relatively straightforward to diagnose and fix. By ensuring that your credentials are properly configured, using IAM roles where possible, and following best security practices, you can avoid this error and keep your AWS applications running smoothly.